Anmation System
A part of a university module on game engine animaiton systems. My goal with this software was to create a system with the best possible API and with minimum depencance on platform being used, so that the module could be easily inserted into any project.
What was created was a pretty powerful 2D sprite & skeletal, and 3D blend-node based skeletal animation system, with suport for IK as well as a methods for loading in the animation data.
The system was created to be used with a propriety C++ engine, which is what is running the demonstration gifs, but care was taken to minimise dependancy on this framework.
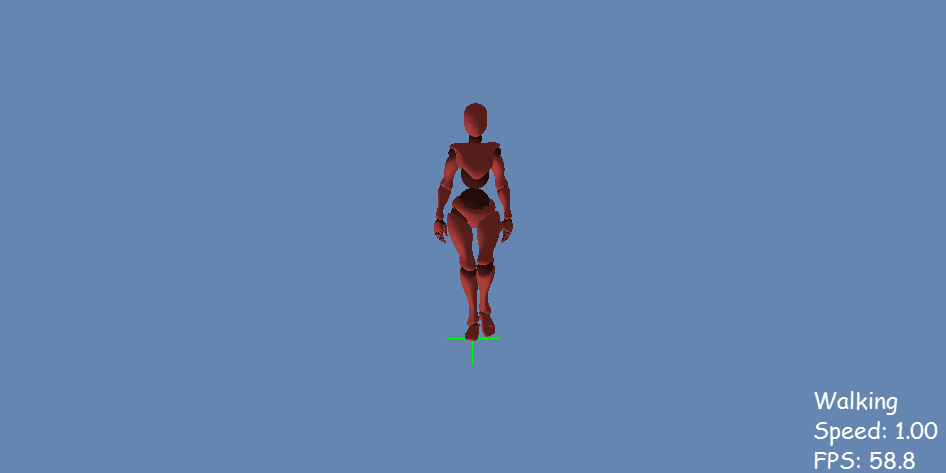
There were two areas of intrest for me in this project. The first being
AnimationSystem::PureResult AnimatedMeshApp::LoadMeshAndAnimation()
{
// create an animated object, returns Value/Error discriminated union
auto createPlayerResult = animation_system_->CreateAnimatedObject("Player",
"xbot/xbot.scn") ;
// early return the error if there is one
if(createPlayerResult.IsError())
return createPlayerResult.ToPureResult();
// move player from result to member variable
player_ = createPlayerResult.Take();
// load the animations, configure with delegates, return early in the case of error
if(auto animationResult =
animation_system_->CreateAnimationFor(*player_,
"Walk","xbot/xbot@walking_inplace.scn","",
[](IAnimatorConfig& animPlayer)
{
animPlayer.SetAnimationTime(0);
animPlayer.SetLooping(true);
});
animationResult.IsError())
{
return animationResult;
}
if(auto animationResult =
animation_system_->CreateAnimationFor(*player_,
"Run","xbot/xbot@running_inplace.scn","",
[](IAnimatorConfig& animPlayer)
{
animPlayer.SetAnimationTime(0);
animPlayer.SetLooping(true);
});
animationResult.IsError())
{
return animationResult;
}
// set the player's animation state
if(auto result = player_->Animator().SetAnimation("Walk"); result.IsError())
return result;
// return OK
return AnimationSystem::PureResult::OK();
}